The code below will be used to add products to a visitor’s cart when they click “Add to cart” in a recommendation widget (some widgets have this feature).
Setting this correctly will enable you to use the “quick add to cart” feature when you recommend an item to a visitor, making it easy to quickly and conveniently add one or more products to their cart, without needing to go to their separate product pages. For Magento, Shopify, etc. you may ask your account manager to provide existing templates that can work with this functionality, out of the box.
Please, edit this code template at your account product interactions settings:
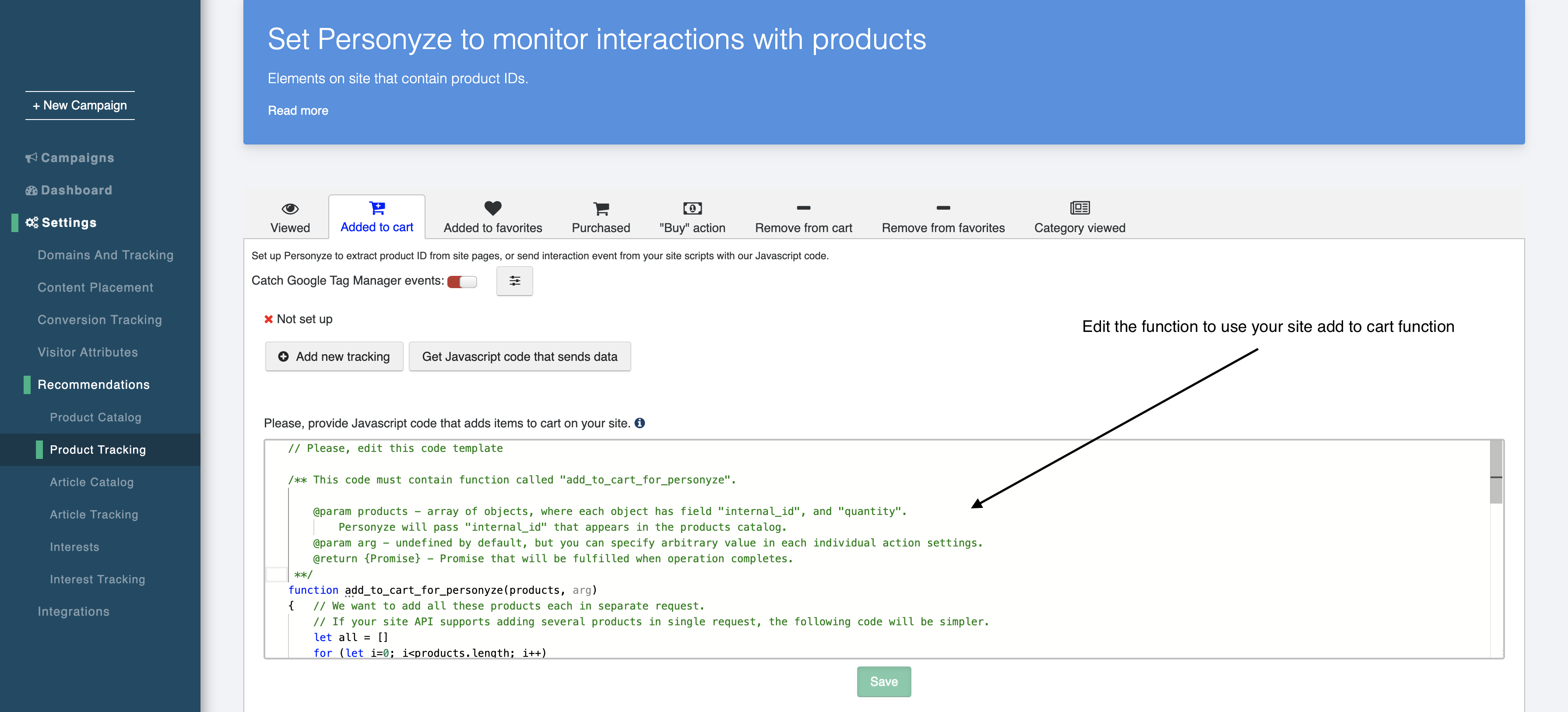
You can change the variable passes to your site function from the widget settings
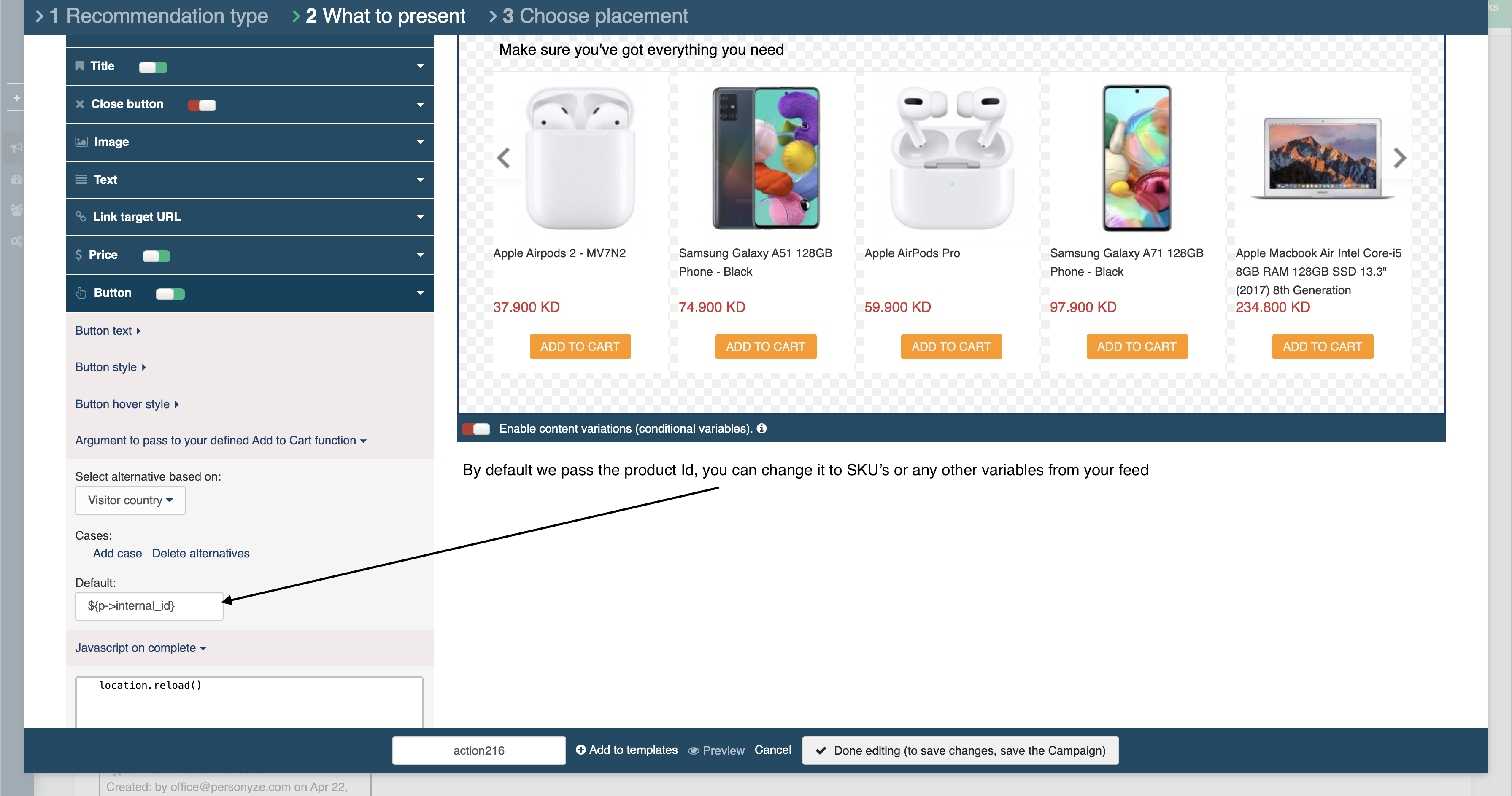
/** This code must contain the function called “add_to_cart_for_personyze”.
@param products – array of objects, where each object has field “internal_id”, and “quantity”.
Personyze will pass “internal_id” that appears in the product catalog.
@param arg – undefined by default, but you can specify an arbitrary value in each individual action settings.
@return {Promise} – Promise that will be fulfilled when the operation completes.
**/
function add_to_cart_for_personyze(products, arg)
{ // We want to add all these products each in separate request.
// If your site API supports adding several products in single request, the following code will be simpler.
let all = []
for (let i=0; i<products.length; i++)
{ // Replace this with your actual request.
all[i] = fetch
( ‘/cart/add’,
{ mode: ‘cors’,
credentials: ‘include’,
method: ‘POST’,
body: ‘product=’+products[i].internal_id+’&qty=’+products[i].quantity
}
).then
( response =>
{ if (!response.ok)
{ throw new Error(response.statusText)
}
return response.text()
}
)
}
// Done.
returnPromise.all(all)
// Or, if your response contains current cart state, it’s good to update Personyze’s state.
returnPromise.all(all).then
( responses =>
{ personyze.push([‘Products Removed from cart’])
for (let response of responses)
{ response = JSON.parse(response) // or use response.json() in fetch
for (let product of response.currently_in_cart)
{ personyze.push([‘Product Added to cart’, product.id])
}
}
}
)
}